Java项目:户政电子档案管理平台(java+SpringBoot+Vue+axios+Mybaits+mysql)
源码获取:俺的博客首页 "资源" 里下载!
项目介绍
Springboot户政电子档案管理平台系统为前后端分离的项目,
主要分为管理员、用户两种角色。
管理员角色主要功能如下:
首页
系统用户管理:管理员用户查询、添加、修改、删除;
单位管理:单位查询、添加、修改、删除;
部门管理:部门查询、添加、修改、删除;
岗位管理:岗位查询、添加、修改、删除;
用户管理:用户查询、添加、修改、删除;
案卷类别管理:案卷类别查询、添加、修改、删除;
案卷管理:案卷查询、添加、修改、删除;
案卷目录管理:案卷目录查询、添加、修改、删除;
人口基本管理:人口基本查询、添加、修改、删除;
案卷统计:按案卷类别统计;
人口基本统计:按户口类型统计;
用户主要功能如下:
首页;
个人资料管理:修改个人资料;
案卷类别管理:案卷类别查询;
案卷管理:案卷查询;
案卷目录管理:案卷目录查询;
人口基本管理:人口基本查询;
环境需要
1.运行环境:最好是java jdk 1.8,我们在这个平台上运行的。其他版本理论上也可以。
2.IDE环境:IDEA,Eclipse,Myeclipse都可以。推荐IDEA;
3.硬件环境:windows 7/8/10 1G内存以上;或者 Mac OS;
4.数据库:MySql 5.7/8.0版本均可;
5.是否Maven项目:是;
技术栈
后端:SpringBoot+Mybaits
前端:Vue+axios
后端项目运行 - houduan:
1. 使用Navicat或者其它工具,在mysql中创建对应sql文件名称的数据库,并导入项目的sql文件;
2. 使用IDEA/Eclipse/MyEclipse导入项目houduan,导入成功后请执行maven clean;maven install命令,然后运行;
3. 将项目中application.yml配置文件中的数据库配置改为自己的配置;
4. 控制台提示运行成功后再运行前端项目;
前端项目运行 - houtai:
1.命令行cd进入 前端目录 houtai;
2.执行命令 npm install 下载依赖;
3.执行命令 npm run dev 启动;
4.运行项目,在浏览器中输入地址:http://localhost:9999/
管理员账号、密码:hsg/hsg
用户账号、密码:666/001 其它用户的密码也均为001
登录展示页面:
管理员用户管理页面:
首页展示页面:
部门管理页面:
单位管理页面:
岗位查询管理页面: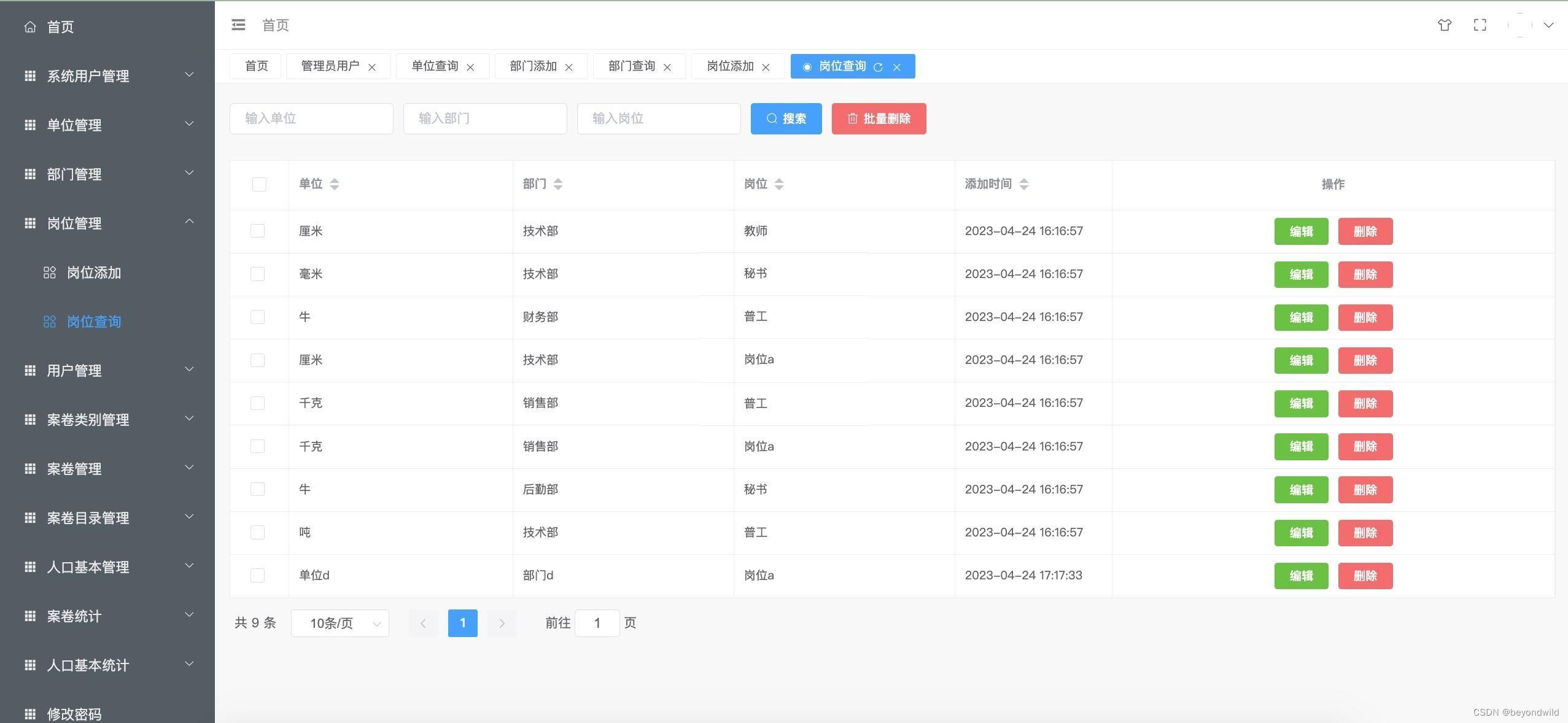
用户查询管理列表:
案卷查询页面: 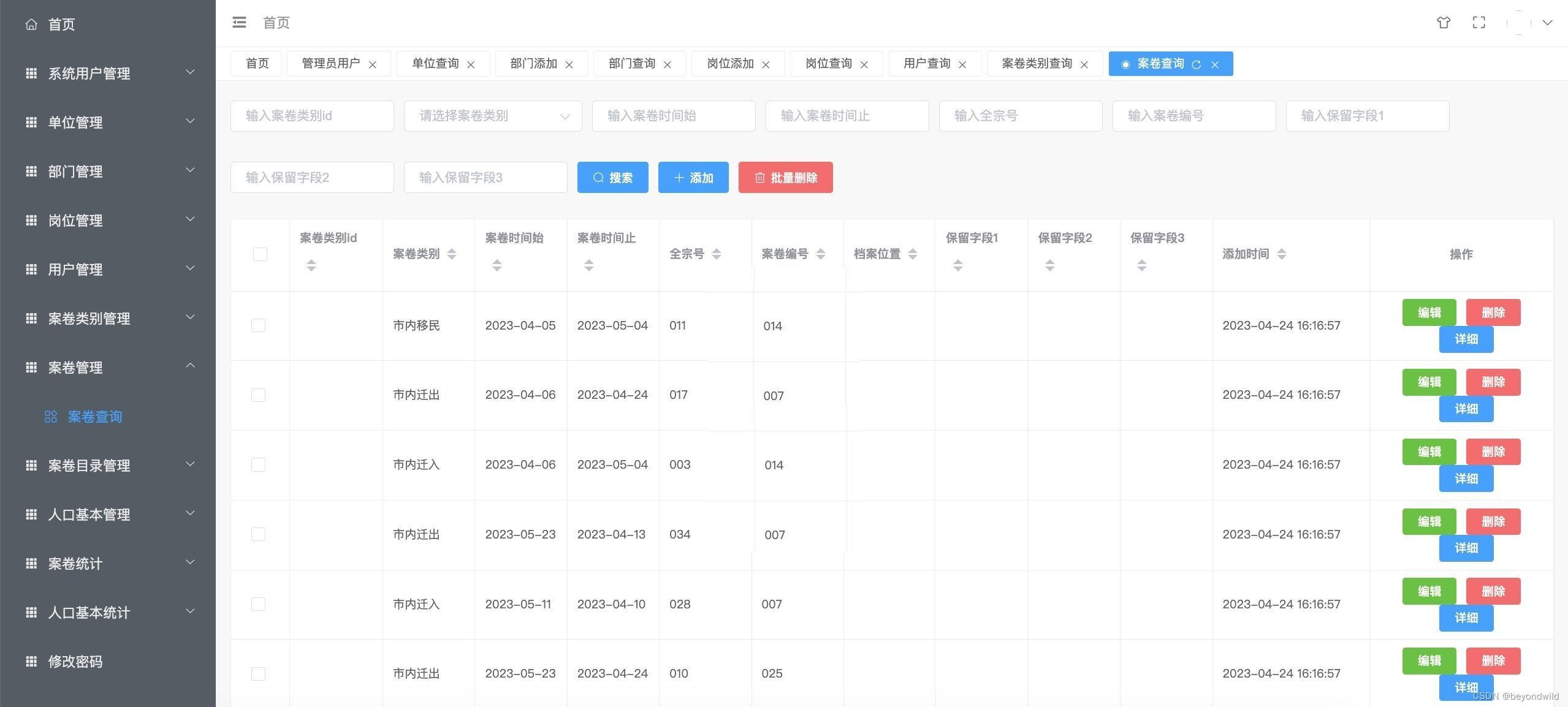
登录管理控制层:
@RestController
@RequestMapping("/login")
public class LoginController {
@Autowired
private StudentEntityMapper studentEntityMapper;
@Autowired
private AdminEntityMapper adminEntityMapper;
@Autowired
private TeacherEntityMapper teacherEntityMapper;
@PostMapping("/login")
public Integer login(@RequestParam(value = "username") String username, @RequestParam(value = "password") String password,
@RequestParam(value = "userType") String userType) {
HttpServletRequest request = ((ServletRequestAttributes) RequestContextHolder.getRequestAttributes()).getRequest();
HttpSession session = request.getSession();
UserTypeEnum userTypeEnum = UserTypeEnum.getEventByCode(userType);
Integer result = 0;
switch (userTypeEnum) {
case ADMIN:
AdminEntityExample example = new AdminEntityExample();
example.or().andUsernameEqualTo(username).andUserpwEqualTo(password);
AdminEntity entity = adminEntityMapper.selectOneByExample(example);
if (null != entity) {
result = 1;
session.setAttribute("loginUserType", UserTypeEnum.ADMIN.getCode());
session.setAttribute("loginUserId", entity.getUserid());
}
break;
case TEACHER:
TeacherEntityExample exampleTea = new TeacherEntityExample();
exampleTea.or().andLoginNameEqualTo(username).andLoginPwEqualTo(password);
TeacherEntity entity2 = teacherEntityMapper.selectOneByExample(exampleTea);
if (null != entity2) {
result = 2;
session.setAttribute("loginUserType", UserTypeEnum.TEACHER.getCode());
session.setAttribute("loginUserId", entity2.getTeaId());
}
break;
case STUDENT:
StudentEntityExample exampleStu = new StudentEntityExample();
exampleStu.or().andLoginNameEqualTo(username).andLoginPwEqualTo(password);
StudentEntity studentEntity = studentEntityMapper.selectOneByExample(exampleStu);
if (null != studentEntity) {
result = 3;
session.setAttribute("loginUserType", UserTypeEnum.STUDENT.getCode());
session.setAttribute("loginUserId", studentEntity.getStuId());
}
break;
default:
return 0;
}
return result;
}
@GetMapping("/getLogin")
public String getCurrentLoginUser() {
HttpServletRequest request = ((ServletRequestAttributes) RequestContextHolder.getRequestAttributes()).getRequest();
HttpSession session = request.getSession();
String loginUser = session.getAttribute("loginUserType").toString();
return loginUser;
}
@RequestMapping("/logout")
public Boolean logout() {
try {
HttpServletRequest request = ((ServletRequestAttributes) RequestContextHolder.getRequestAttributes()).getRequest();
HttpSession session = request.getSession();
session.removeAttribute("loginUserType");
session.removeAttribute("loginUserId");
return true;
} catch (Exception e) {
e.printStackTrace();
}
return false;
}
}
管理员跳转控制层:
@RestController
@RequestMapping("/admin")
public class AdminController {
@Autowired
private AdminEntityMapper adminEntityMapper;
@PostMapping("/add")
public Integer add(@RequestBody AdminEntity entity) {
return adminEntityMapper.insert(entity);
}
@PostMapping(value = "/delete", consumes = "application/json")
public Integer delete(@RequestBody AdminEntity entity) {
return adminEntityMapper.deleteByPrimaryKey(entity.getUserid());
}
@PostMapping("/update")
public Integer update(@RequestBody AdminEntity entity) {
return adminEntityMapper.updateByPrimaryKey(entity);
}
@RequestMapping("/select")
public Layui select(@RequestParam(required = false) String username,@RequestParam(value = "page")Integer page,
@RequestParam(value = "limit")Integer limit) {
AdminEntityExample example = new AdminEntityExample();
if (!StringUtils.isEmpty(username)){
example.or().andUsernameLike("%"+username+"%");
}
example.getOrderByClause();
Long cou = adminEntityMapper.countByExample(example);
return Layui.data(cou.intValue(), adminEntityMapper.selectByExamplePaging(example, page-1, limit));
}
@RequestMapping("/getAdmin")
public AdminEntity getAdmin(@RequestParam(value="userid")Integer userid) {
return adminEntityMapper.selectByPrimaryKey(userid);
}
}
首页管理控制层:
@RestController
@RequestMapping("/first")
public class IndexController {
@Autowired
private IndexTableEntityMapper indexTableEntityMapper;
@PostMapping("/add")
public Integer add(@RequestBody IndexTableEntity entity) {
if (StringUtils.isEmpty(entity.getParentId())) {
entity.setParentId(null);
}
return indexTableEntityMapper.insert(entity);
}
@PostMapping(value = "/delete", consumes = "application/json")
public Integer delete(@RequestBody IndexTableEntity entity) {
return indexTableEntityMapper.deleteByPrimaryKey(entity.getId());
}
@PostMapping("/update")
public Integer update(@RequestBody IndexTableEntity entity) {
return indexTableEntityMapper.updateByPrimaryKey(entity);
}
@RequestMapping("/select")
public Layui select(@RequestParam(required = false) String indexname, @RequestParam(value = "page") Integer page,
@RequestParam(value = "limit") Integer limit) {
IndexTableEntityExample example = new IndexTableEntityExample();
IndexTableEntityExample.Criteria criteria = example.createCriteria();
if (!StringUtils.isEmpty(indexname)) {
criteria.andIndexnameLike("%"+indexname+"%");
}
criteria.andParentIdIsNotNull();
Long cou = indexTableEntityMapper.countByExample(example);
return Layui.data(cou.intValue(), indexTableEntityMapper.selectByExamplePaging(example, page - 1, limit));
}
@RequestMapping("/selectFir")
public List<IndexTableEntity> selectFir() {
IndexTableEntityExample example = new IndexTableEntityExample();
example.or().andParentIdIsNull();
return indexTableEntityMapper.selectByExample(example);
}
@RequestMapping("/selectList")
public List<IndexTableEntity> selectList() {
IndexTableEntityExample example = new IndexTableEntityExample();
example.or().andParentIdIsNotNull();
return indexTableEntityMapper.selectByExample(example);
}
@RequestMapping("/getFirst")
public IndexTableEntity getAdmin(@RequestParam(value = "id") Integer userid) {
return indexTableEntityMapper.selectByPrimaryKey(userid);
}
}
源码获取:俺的博客首页 "资源" 里下载!